Android animations
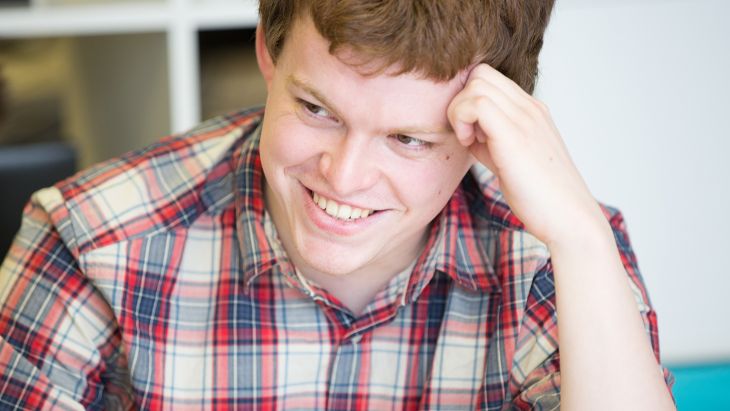
Dazed and confused
Android animations have not always been the easiest task to execute. They were complex and confusing.
Thankfully, things have significantly improved as of late making animations significantly simpler and, to be honest, rather fun to implement.
In this post we'll explore some of the commonly used solutions, which will hopefully inspire you to create even more exciting ones.
Parallax scrolling
Firstly, let's take a look at Parallax Scrolling.
You'll find it surprisingly easy on Android, at least that is if you are willing to use a RecyclerView (which I would definitely recommend).
RecyclerView has a nice easy OnScrollListener which gives you, in the onScrolled method, the delta x and y of the scroll.
This means that you know how much the RecyclerView has scrolled, hence making it nice and easy to move something else using those amounts.
In this example I have scrolled the ToolBar by half the amount that the RecyclerView scrolled.
To achieve this I just use the View.setTranslationY
method to translate the ToolBar by the desired amount.
Using a quick if statement for up and down (dy > 0)
and Math.max()
and Math.min()
to stop the ToolBar moving too far away, we get this wonderful parallax affect.
Quick and easy to implement, but adds a mark of quality above other apps.
The code
final View parallaxView = rootView.findViewById(R.id.parallax_bar); if (null != parallaxView) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB) { mRecyclerView.addOnScrollListener(new RecyclerView.OnScrollListener() { @TargetApi(Build.VERSION_CODES.HONEYCOMB) @Override public void onScrolled(RecyclerView recyclerView, int dx, int dy) { super.onScrolled(recyclerView, dx, dy); Int max = parallaxView.getHeight(); if (dy > 0) { parallaxView.setTranslationY(Math.max(-max, parallaxView.getTranslationY() - dy / 2)); } else { parallaxView.setTranslationY(Math.min(0, parallaxView.getTranslationY() - dy / 2)); } } }); } }
Animating Transistions
Another simple way to give the user a little more of a 'wow' factor is to change how the screen populates itself.
For the best effect it's worth ensuring the transitions feel natural and not overused or over elaborate. You don't want a screen to have things flying in from all over the place, 90s powerpoint style.
It doesn't have to be too much work to achieve these transitions.
First you need to create the entering and returning transitions in an xml file.
Use target android:targetId="@id/toolbar"
to tell the system which view the transition is talking about within a transition type like slide android:slideEdge="bottom"
.
Then create a style for the opening activity setting the entering and returning transitions.
The final piece of magic is to startActivity
in the slightly different way specified below.
You'll now have subtle but effective transitions that your users will love.
The code
enter_transition.xml
<transitionSet xmlns:android="http://schemas.android.com/apk/res/android" android:transitionOrdering="together" android:duration="250"> <fade xmlns:android="http://schemas.android.com/apk/res/android"> <targets> <target android:excludeId="@android:id/statusBarBackground"/> <target android:excludeId="@android:id/navigationBarBackground"/> </targets> </fade> <slide android:slideEdge="top"> <targets> <target android:targetId="@id/toolbar" /> </targets> </slide> <slide android:slideEdge="bottom"> <targets> <target android:targetId="@id/detail_additional_pane" /> </targets> </slide> </transitionSet>
return_transition.xml
<transitionSet xmlns:android="http://schemas.android.com/apk/res/android" android:transitionOrdering="together" android:duration="250"> <fade> <targets> <target android:excludeId="@android:id/statusBarBackground" /> <target android:excludeId="@android:id/navigationBarBackground" /> </targets> </fade> <slide android:slideEdge="bottom"> <targets> <target android:targetId="@id/detail_additional_pane" /> </targets> </slide> </transitionSet>
styles.xml
<style name="AppTheme.DetailsTransition" parent="@style/AppTheme"> <item name="android:windowContentTransitions">true</item> <item name="android:windowEnterTransition">@transition/enter_transition</item> <item name="android:windowReturnTransition">@transition/return_transition</item> </style>
Shared Element Transitions
Adding a shared element is nice way of exciting the user. It is also a great tool for providing continuity between pages and drawing the users eye toward said element.
A great use case is in lists which shares an icon/picture with the detail view you get once clicked.
To implement you need to tell the system which two elements are linked by setting android:transitionName=”@string/transition_name”
in the xml (you can also set it programmatically) on the two elements.
Then to make sure that the animation is smooth you should use a tool called supportPostponeEnterTransition
which will basically halt everything while your page loads.
That being said you should not be doing network calls in that time, as they could take a long time.
This delay is designed for waiting until the page has been drawn primarily.
Then simply start the activity as below, then run and watch your elements fly.
The code
Set on the shared elements:
android:transitionName=”@string/transition_name” ViewCompat.setTransitionName(imageView, getString(@string/transition_name));
Set in onActivityCreated:
Activity.supportPostponeEnterTransition();
Set when your new page has loaded (for example in onLoadFinished):
Activity.supportStartPostponedEnterTransition();
To start activity:
ActivityOptionsCompat activityOptions = ActivityOptionsCompat.makeSceneTransitionAnimation(this, new Pair(vh.mIconView, getString(R.string.detail_icon_transition_name))); ActivityCompat.startActivity(this, intent, activityOptions.toBundle()); ,>
For more animations...
This material has been sourced primarily from the Udacity course Advanced Android App Development which I would highly recommend browsing.
Looking for something else?
Search over 400 blog posts from our team
Want to hear more?
Subscribe to our monthly digest of blogs to stay in the loop and come with us on our journey to make things better!