How to get your notifications noticed (on Android)
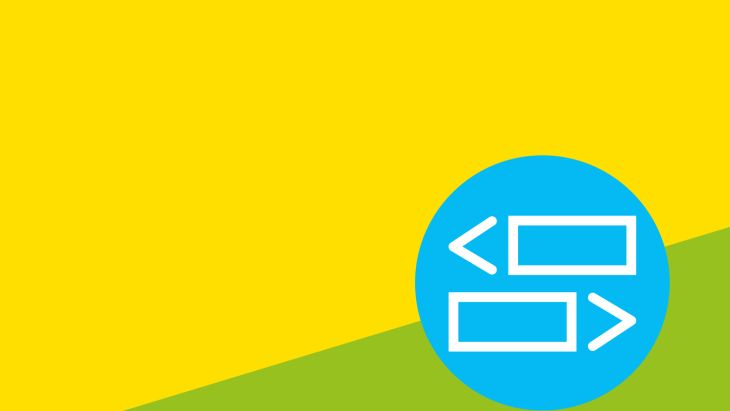
Godsend or spam?
Depending on how you view them, notifications are either those helpful prompts delivered straight to your home screen or those annoying, spammy pop-up messages which repeatedly interrupt your daily life.
Whichever perspective you have, most of us can no longer live without them and developers constantly need to learn how and when to best deploy them.
Android has been making significant improvements to notifications and they continue to be a key focus through the developing of Android Nougat. We’ll bear this in mind as we outline some key guidelines to deploying the most user-focused notifications and how to build them.
Does the user need this?
Before you start diving in and displaying notifications all over the shop, first STOP! Have a think; does the user need to know this right now? How can we simply and effectively serve the user with this notification?
For example, a messaging application should show a notification when the user gets a new message, possibly with a quick reply action. But, a notification saying a friend has started playing a game (good grief) might not be the most useful piece of information for the user right now (or ever).
Displaying user focused notifications benefits the developer too. If the user constantly receives spammy notifications from your app, they will start to ignore them, or even worse block them. Which means none of the information you want to give the user will be seen, including your best marketing.
Here's how
Simple notifications
NotificationCompat.Builder mBuilder = new NotificationCompat.Builder(this) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("My notification") .setContentText("Hello World!"); Intent resultIntent = new Intent(this, ResultActivity.class); TaskStackBuilder stackBuilder = TaskStackBuilder.create(this); stackBuilder.addParentStack(ResultActivity.class); stackBuilder.addNextIntent(resultIntent); PendingIntent resultPendingIntent = stackBuilder.getPendingIntent(0, PendingIntent.FLAG_UPDATE_CURRENT ); mBuilder.setContentIntent(resultPendingIntent); NotificationManager mNotificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); mNotificationManager.notify(mId, mBuilder.build());
This code will show a notification to the user with notificaition_icon as the icon, “My notification” as a title and “Hello World!” as the message. When the user taps on the notification they will be taken to ResultActivity
.
Notifications that delight the user
Now we can look at some of the more interesting parts of notifications.
setLargeIcon(Bitmap icon)
With this method, you can set an icon to be displayed when the notification is viewed in a bigger context.
Examples of a bigger context would be when you open the notifications menu by swiping down from the top of the screen, or if your notification is displayed in a heads up (overlays on top of the current screen).
Using this option can make the notification feel more personal e.g if you display a message from a friend and you set the large icon to the friend's profile picture.
setColor(@ColorInt int argb)
This method allows you to set an accent colour for the notification. This helps make it more personalised to your app.
setPriority(int pri)
This allows you to prioritise your notification, not just within your own apps notifications but the whole system.
This is often a source for blocking notifications, as users do not want low priority notifications to be given the highest priority. See the docs for more details .
setLights(@ColorInt int argb, int onMs, int offMs)
Now this is a fun option. You can set the colour and pulse timings of the notification light.
Setting this will help the users who use the pulse light and it gives your application a more distinguishable place within that.
setSound(Uri sound)
A classic, yet often gets missed - Set the sound to play with your notification.
This is a great opportunity to bring your brand to life with a sound which is delightful and unique. Be careful to not create something your user will begin to hate, and maybe offer some options within your settings.
addPerson(String uri)
This option is useful for message applications.
It enables you to associate the notification with a contact on the user's phone. This means that if the user has interruption filters, or some other settings your application will comply, again making life better for the user.
You can even pass a phone number into this, just prefix the phone number with “tel:”
setPublicVersion(Notification n)
This option allows you to set a different notification for a public situation e.g. the lock screen.
Simply build a different notification (as per normal) and then set it with this method. This should be used in conjunction with setVisibility(int visibility).
addAction()
This option allows you to add custom actions/buttons to the notification. This could be anything from media playback options or a delete action on an email.
Don’t be tempted to use this to provide an action which should just be used when the user clicks on the notification.
On the same note, you don’t need an action for removing the notification. The user can already do that by swiping. But use the action to give the user a quick option of doing something they continually do which is relevant to the notification content.
In summary
There are many other options available, so please take a look at the NotificationCompat.Builder
class (hint: use auto-complete for a quick way to see the options in code).
Make sure you take a look at the Android docs and happy notifying.
Looking for something else?
Search over 400 blog posts from our team
Want to hear more?
Subscribe to our monthly digest of blogs to stay in the loop and come with us on our journey to make things better!