Mockk.io - our new mocking framework
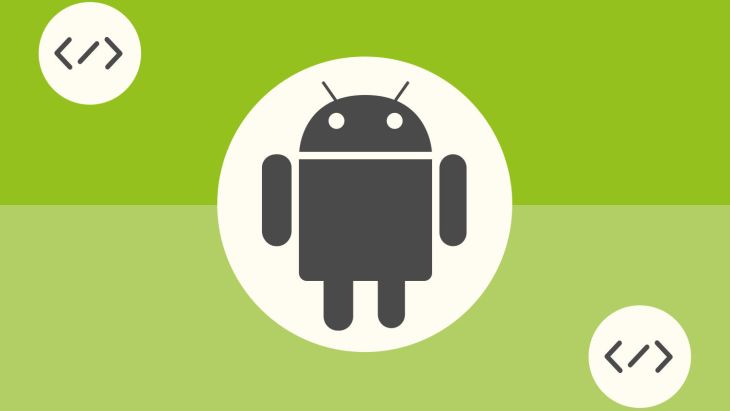
What is mockk.io and why have we adopted it in our team?
Mockk is a mocking framework built for Kotlin. Like Mockito, Mockk allows you to create and stub objects within your test code.
Mocking objects allows you to test in isolation other objects. Any dependency of the object under test can be mocked to provide fixed conditions, thus ensuring specific and stable tests.
Mockito is a common framework used by Java developers and is very powerful. But it does have some annoyances when used with Kotlin. Mockk, being specifically designed for Kotlin, is a more pleasant experience.
How to use it
With Mockito this is how you mocked an object:
import static org.mockito.Mockito.mock; MyClass mockedClass = mock(MyClass.class);
With Mockk it looks like this:
import io.mockk.mockk val mockedClass = mockk<MyClass>()
As you can see these are almost identical, except for the difference in languages.
It’s the same story across the board, from spying to verifying. They are all very similar.
Why we switched
Mockk supports some important language features within Kotlin.
Final by default
In contrast to Java, Kotlin classes (and functions) are final by default. This means Mockito requires extra configuration to enable it to work (and only in some cases). Whereas, Mockk can work around this using inline class transformation.
Chained mocking
With Mockk you can chain your mocking, meaning you can provide concise and clear tests.
val mockedClass = mockk<MyClass>() every { mockedClass.someMethod().someOtherMethod() } returns "Something"
Object mocking
Kotlin objects map to Java statics. Mockito alone doesn't support mocking of statics. There are other frameworks you can combine with Mockito, but Mockk provides this out of the box.
mockObject(MyObject) every { MyObject.someMethod() } returns "Something"
Hierarchical mocking
While mocking out behaviour, you can create mocks and define their behaviour too.
val mockedClass = mockk<MyClass>() every { mockedClass.someMethod() } returns mockk { every { someOtherMethod() } returns "Something" }
Extension functions
Since extension functions map to Java statics, again Mockito doesn't support mocking them. With Mockk, you can mock them without any extra configuration.
Multiplatform
Mockk is a Kotlin Multiplatform framework and they plan to support other platforms. Currently, the JVM is the only supported platform, but I'm excited to see this progress in the future.
Summary
Mockk provides a more Kotlin-friendly approach to mocking. It gives a familiar API while supporting the more complex Kotlin'isms.
Looking for something else?
Search over 400 blog posts from our team
Want to hear more?
Subscribe to our monthly digest of blogs to stay in the loop and come with us on our journey to make things better!