Calling RESTful APIs with Retrofit
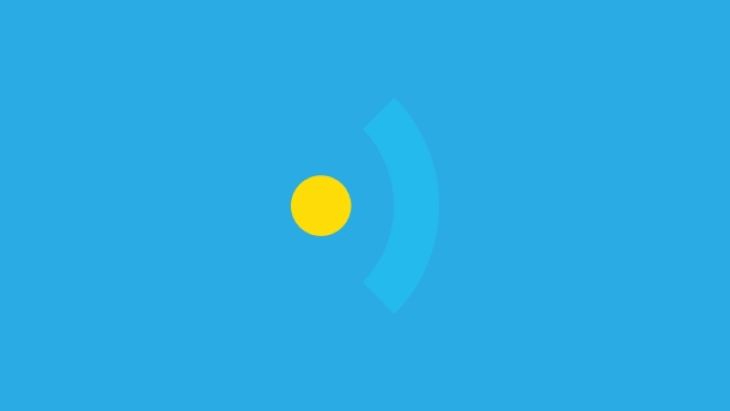
Less boilerplate code
Recently in one of my Android projects, I used a library called Retrofit which is “a type-safe REST client for Android and Java”.
It is a small library and deceptively simple to use but has the potential to save you from writing a lot of [boilerplate] code.
No app is an island
When developing an app, sooner or later you are going to want to make contact with the outside world to fetch some data or information.
Whether you are looking for the current weather, the latest stock price or a twitter feed there will normally be a web service that you can connect with.
A common approach for web service providers is to create RESTful APIs. These are published at a base URI and use standard HTTP methods (like GET, PUT, POST or DELETE) for receiving and sending data.
Calling RESTFul APIs the traditional way
In the past I would have probably created something like this pseudocode:
1. Open a connection to the API
URL connectionURL = new URL(“https://api.github.com/users/brightec/repos”); HttpURLConnection urlConnection = (HttpURLConnection) connectionURL.openConnection(); urlConnection.setRequestMethod(“GET”); int status = urlConnection.getResponseCode(); if (status != 200) { // Do some error handling! return; }
2. Read the HTTP Response from the API
InputStream in = new BufferedInputStream(urlConnection.getInputStream()); InputStreamReader reader = new InputStreamReader(in); BufferedReader bufferedReader = new BufferedReader(reader); StringBuilder builder = new StringBuilder(); String line = bufferedReader.readLine(); while (line != null) { builder.append(line); line = bufferedReader.readLine(); } String httpResponse = builder.toString();
3. Finally do something with the HTTP response, probably converting JSON into Java classes
JsonObject jsonResponse = new JsonParser().parse(httpResponse).getAsJsonObject(); Gson gson = GsonBuilder().create; Repo[] repos = gson.fromJson(jsonResponse, Repo[].class);
Calling RESTFul APIs the Retrofit way
Fortunately, there is an easier way. In three simple steps follow the Retrofit way:
1. Define the API web service as an interface using Retrofit annotations
public interface GitHubService { @GET("/users/{user}/repos") List listRepos(@Path("user") String user); }
2. Use Retrofit’s RestAdapter to create an implementation of your interface
RestAdapter restAdapter = new RestAdapter.Builder() .setEndpoint("https://api.github.com") .build(); GitHubService service = restAdapter.create(GitHubService.class);
3. Call the generated service to make the HTTP request to the API
List repos = service.listRepos("brightec");
Using Retrofit, most of the heavy lifting is done for you. Most excitingly, this is just the beginning of what it can do.
Find out more about how to use Retrofit here.
Looking for something else?
Search over 400 blog posts from our team
Want to hear more?
Subscribe to our monthly digest of blogs to stay in the loop and come with us on our journey to make things better!